- Big 0 Notation Cheat Sheet
- Big-o Algorithm Complexity Cheat Sheet Pdf
- Algorithm Time Complexity Cheat Sheet
Below are the Big O performance of common functions of different Java Collections. |
List | Add | Remove | Get | Contains | Next | Data Structure |
---------------------|------|--------|------|----------|------|--------------- |
ArrayList | O(1) | O(n) | O(1) | O(n) | O(1) | Array |
LinkedList | O(1) | O(1) | O(n) | O(n) | O(1) | Linked List |
CopyOnWriteArrayList | O(n) | O(n) | O(1) | O(n) | O(1) | Array |
Set | Add | Remove | Contains | Next | Size | Data Structure |
----------------------|----------|----------|----------|----------|------|------------------------- |
HashSet | O(1) | O(1) | O(1) | O(h/n) | O(1) | Hash Table |
LinkedHashSet | O(1) | O(1) | O(1) | O(1) | O(1) | Hash Table + Linked List |
EnumSet | O(1) | O(1) | O(1) | O(1) | O(1) | Bit Vector |
TreeSet | O(log n) | O(log n) | O(log n) | O(log n) | O(1) | Red-black tree |
CopyOnWriteArraySet | O(n) | O(n) | O(n) | O(1) | O(1) | Array |
ConcurrentSkipListSet | O(log n) | O(log n) | O(log n) | O(1) | O(n) | Skip List |
Queue | Offer | Peak | Poll | Remove | Size | Data Structure |
------------------------|----------|------|----------|--------|------|--------------- |
PriorityQueue | O(log n) | O(1) | O(log n) | O(n) | O(1) | Priority Heap |
LinkedList | O(1) | O(1) | O(1) | O(1) | O(1) | Array |
ArrayDequeue | O(1) | O(1) | O(1) | O(n) | O(1) | Linked List |
ConcurrentLinkedQueue | O(1) | O(1) | O(1) | O(n) | O(n) | Linked List |
ArrayBlockingQueue | O(1) | O(1) | O(1) | O(n) | O(1) | Array |
PriorirityBlockingQueue | O(log n) | O(1) | O(log n) | O(n) | O(1) | Priority Heap |
SynchronousQueue | O(1) | O(1) | O(1) | O(n) | O(1) | None! |
DelayQueue | O(log n) | O(1) | O(log n) | O(n) | O(1) | Priority Heap |
LinkedBlockingQueue | O(1) | O(1) | O(1) | O(n) | O(1) | Linked List |
Map | Get | ContainsKey | Next | Data Structure |
----------------------|----------|-------------|----------|------------------------- |
HashMap | O(1) | O(1) | O(h / n) | Hash Table |
LinkedHashMap | O(1) | O(1) | O(1) | Hash Table + Linked List |
IdentityHashMap | O(1) | O(1) | O(h / n) | Array |
WeakHashMap | O(1) | O(1) | O(h / n) | Hash Table |
EnumMap | O(1) | O(1) | O(1) | Array |
TreeMap | O(log n) | O(log n) | O(log n) | Red-black tree |
ConcurrentHashMap | O(1) | O(1) | O(h / n) | Hash Tables |
ConcurrentSkipListMap | O(log n) | O(log n) | O(1) | Skip List |
Official Big-O Cheat Sheet Poster. Millions of unique designs by independent artists. Find your thing. Saved by Redbubble. Python Programming Computer Programming Computer Science Computer Tips Computer Humor Computer Coding Gaming Tips Linux Java Cheat Sheet. View Big-O Algorithm Complexity Cheat Sheet.pdf from CSE 3 at Engineering College.
commented Feb 7, 2021
You've mixed data structures between LinkedList and ArrayDequeue in the Queue section. |
commented Mar 11, 2021
Big 0 Notation Cheat Sheet
Do these figures still hold in the new Java versions? If not, then I would suggest adding the Java-version these performances were measured at. I presume by and large the performance does not change dramatically though. |

Big-o Algorithm Complexity Cheat Sheet Pdf
commented Apr 21, 2021
Algorithm Time Complexity Cheat Sheet
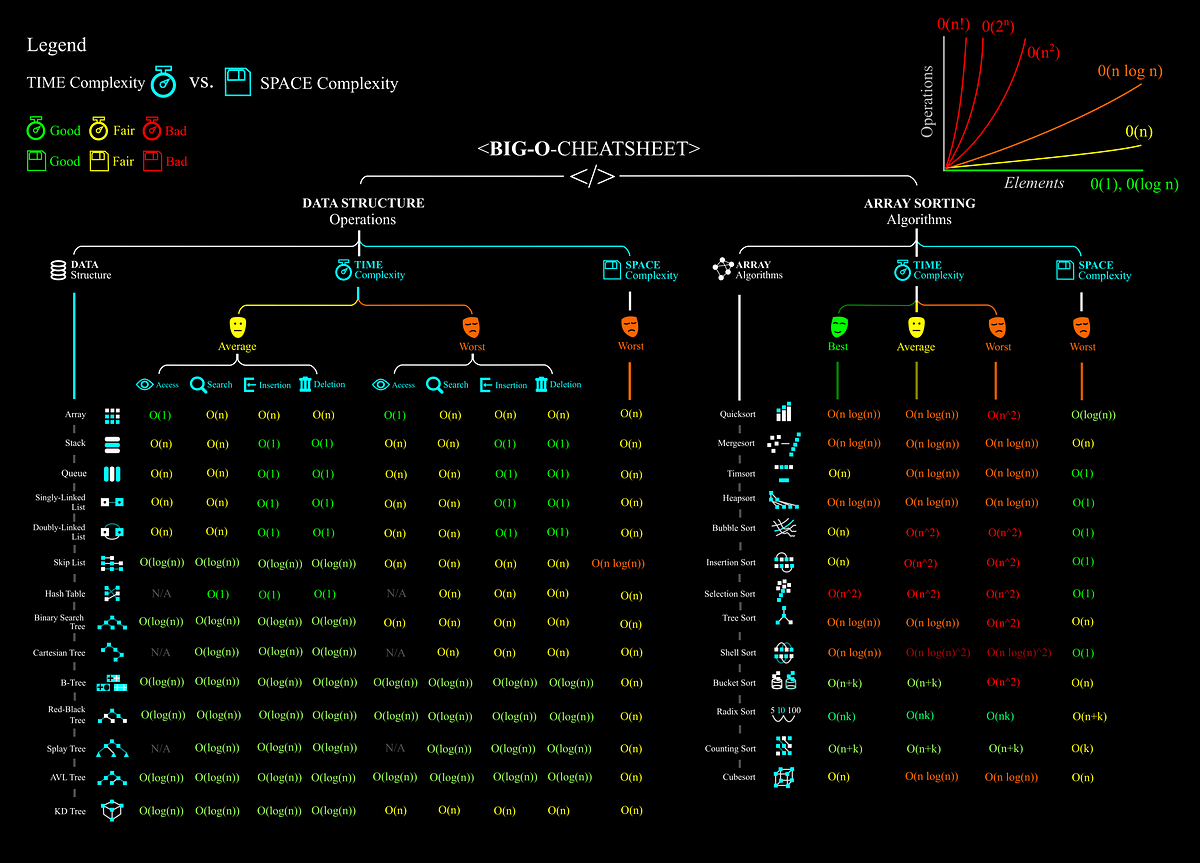
I highly doubt this was measured. It's probably inferred from the implementation. And I doubt the complexity of the implementations changed. Most algorithms have been around for a long time. |
